Assignment Brief
As part of the formal assessment for the programme, you are required to submit an Introduction to Programming assignment. Please refer to your Student Handbook for full details of the programme assessment scheme and general information on preparing and submitting assignments. The assignment brief will specifically give details and instructions for the assignment.
Module grade: Coursework 100%
Learning outcomes:
After completing the module, you should be able to:
- LO1: Develop an understanding of data structures and programming techniques in the context of a programming language.
- LO2: Demonstrate an understanding of how programs are developed, i.e., from concept to development and testing.
- LO3: Demonstrate an ability to write programs using appropriate structure and language rules.
Graduate attributes
- Discipline Expertise: Knowledge and understanding of the chosen field. Possess a range of skills to operate within this sector, have a keen awareness of current developments in working practice being well positioned to respond to change.
All learning outcomes must be met to pass the module.
Guidance
Your assignment should include a title page containing your student number, the module name, the submission deadline, and the exact word count of your submitted document; the appendices if relevant; and a reference list in AU Harvard system(s). You should address all the elements of the assignment task listed below. Please note that tutors will use the assessment criteria set out below in assessing your work.
You must not include your name in your submission because Arden University operates anonymous marking, which means that markers should not be aware of the identity of the student. However, please do not forget to include your STU number.
Maximum word count: 3000 words
Please refer to the full word count policy which can be found in the Student Policies section here: Arden University | Regulatory Framework
Note: The word count includes everything in the main body of the assessment (including in-text citations and references). The word count excludes numerical data in tables, figures, diagrams, footnotes, reference list, and appendices. All other printed words ARE included in the word count.
Students who exceed the word count up to a 10% margin will not be penalised. Students should note that no marks will be assigned to work exceeding the specified limit once the maximum assessment size limit has been reached.
Do You Need Assignment of This Question
Scenario
A small retailer would like a Java program for managing their stock. Some items may be withdrawn from the stock while new items may be added periodically. The retailer wants a convenient way to store this information and manage it effectively. Any errors in the management of the stock should be avoided as they can create issues for sales.
During the working week, when items are sold, the stock is updated and reorders are placed daily to restock items that have fallen below their threshold. The value for the threshold indicated in Table 1 indicates the minimum current stock level needed below which a re-order is triggered. The reorder quantity indicated in Table 1 is used for each item being reordered.
Stock table with typical items.
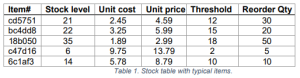
The retailer would like the programme to display the following menu:
Stock control menu
1. Enter stock data
2. Enter sales data
3. Exit
Figure 1. Menu to be displayed for the program.
Typical sales data for a day has been provided in Table 2
Item | Qty Sold |
---|---|
bc4dd8 | 8 |
18b050 | 20 |
c47d16 | 4 |
6c1af3 | 5 |
Based on the stock from Table 1 and sales from Table 2, reorder list would be tabulated as illustrated:
Item | bc4dd8 | 18b050 | 6c1af3 |
---|---|---|---|
Current level | 14 | 15 | 9 |
Threshold | 45 | 18 | 10 |
Reorder Qty | 20 | 50 | 10 |
Unit cost | 3.25 | 1.89 | 5.78 |
Subtotal | 65.00 | 94.50 | 57.80 |
Total cost 217.30
Assignment Task
Task 1
- Provide a flowchart and pseudo-code to display the menu as in Figure 1. Once an option is selected and the code for the option executed, the menu should be displayed again. The program should terminate only when the option for exit is selected.
- a) Explain how you have validated user input (choice selection) and checked for any possible logical errors, while justifying the data types you have used.
- b) Flowchart and pseudocode should use standard conventions and format such as indentations, capitalisation, descriptive names for variables/methods, keywords, operators etc.
- c) The program should take the user input for menu options and execute the appropriate method. When option 1 is selected the method ‘StockData’ should be executed. When option 2 is selected the method ‘SalesData’ should be executed.
- d) At this stage each method should only have a print statement such as ‘Enter current stock data for each item’ or ‘Enter sales completed for the day’. No actual choices for the items in stock or sales values are required. The complete Java methods for the selection of option 1 and option 2 will need to be written in task 2 and task 3 respectively.
- Write a Java program based on the algorithm with comments to illustrate the program flow.
- a) The program should take the user input for menu options and execute the appropriate method.
- Test your program by displaying the menu and selecting the various options when the menu is displayed. Provide screenshots for the output of the program showing the correct execution of all menu options.
- Provide the Java code for this task in the Appendix as text for verification.
Task 2
In this task you will write the algorithm, code and test the working of the method ‘StockData’. You must also create a suitable data structure to store the details of the stock items to be provided with their current stock among other details. This data structure should be accessible to read and write in both task 2 and task 3.
- Provide a flowchart and pseudo-code for when option 1 is selected.
- a) The algorithm should demonstrate the acceptance of the user input for the items in stock. The algorithm should accept the data from Table 1, along with appropriate data for an additional 5 items of your choice.
- b) After entering all the stock data, the total cost of the stock should be displayed. e.g. for the stock data in Table 1, the message to be displayed would be ‘The total cost of stock is 328.52’.
- c) Then the menu should be displayed for further user selection.
- d) Explain how you have validated user input (choice selection) and checked for any possible logical errors, while justifying the data types you have used.
- e) Flowchart and pseudocode should use standard conventions and format such as indentations, capitalisation, descriptive names for variables/methods, keywords, operators etc.
- f) The subroutine should take user input from the keyboard for the choices and data entry.
- g) The user input for items in stock should be stored using a suitable data structure.
- Write a Java method ‘StockData’ based on the algorithm with comments to illustrate the program flow.
- a) The method should employ only fixed arrays where necessary – dynamic arrays (i.e. ArrayList, vector, HashMap, etc.) are not to be used.
- You will need to enter all the stock data from Table 1, and an additional 5 new items with appropriate stock data values of your choice. All this data should be entered using the keyboard as user input.
- Test your program by embedding the complete Java code for the ‘StockData’ method in the program written in task 1.
- Provide screenshots for outputs of the Java method for the user input you have used.
- Provide the Java code for this task in the Appendix as text for verification.
Buy Answer of This Assessment & Raise Your Grades
Task 3
In this task you will write the algorithm, code and test the working of the method ‘SalesData’. You will need to use the data stored in task 2 to complete task 3.
- Provide a flowchart and pseudo-code for when option 2 is selected.
- a) The algorithm should take the sales data from Table 2, with appropriate sales values for the additional 5 items you entered in task 2.
- b) Once the sales data is entered, the total sales amount should be displayed. e.g. for the data in Table 1 and Table 2 the message to be displayed would be ‘The total sales amount is 206.83’. Then the reorder list should be displayed as in Table 3.
- c) Then the menu should be displayed for further user selection.
- d) Explain how you have validated user input (choice selection) and checked for any possible logical errors, while justifying the data types you have used.
- e) Flowchart and pseudocode should use standard conventions and format such as indentations, capitalisation, descriptive names for variables/methods, keywords, operators etc.
- Write a Java method ‘SalesData’ based on the algorithm with comments to illustrate the program flow.
- a) The method should employ only fixed arrays where necessary – dynamic arrays (i.e. ArrayList, vector, HashMap, etc.) are not to be used.
- You will need to enter all the sales data from Table 2, and appropriate sales values of your choice for the additional 5 items you entered in Task 2. All this data should be entered using the keyboard as user input.
- Test your program by embedding the complete Java code for the ‘SalesData’ method in the program written in task 1.
- Provide screenshots for outputs of the Java method.
- Provide the full Java code in the Appendix as text for verification. At this stage the program should include the code for the menu display and the completed methods ‘StockData’ and ‘SalesData’.
Assessment Criteria (Learning objectives covered – all.
Level 4 is the first stage on the student journey into undergraduate study. At Level 4 students will be developing their knowledge and understanding of the discipline and will be expected to demonstrate some of those skills and competences. Student are expected to express their ideas clearly and to structure and develop academic arguments in their work. Students will begin to apply the theory which underpins the subject and will start to explore how this relates to other areas of their learning and any ethical considerations as appropriate. Students will begin to develop self-awareness of their own academic and professional development.
Grade Mark Bands | Generic Assessment Criteria |
---|---|
First (1) 80%+ | Outstanding performance which demonstrates the ability to analyse the subject area and to confidently apply theory whilst showing awareness of any relevant ethical considerations. The work shows an outstanding level of competence and confidence in managing appropriate sources and materials, initiative and excellent academic writing skills and professional skills (where appropriate). The work shows originality of thought. |
70-79% Excellent | Excellent performance which demonstrates the ability to analyse the subject and apply theory whilst showing some awareness of any relevant ethical considerations. The work shows a high level of competence in managing sources and materials, initiative and excellent academic writing skills and professional skills (where appropriate). The work shows originality of thought. |
Upper second (2:1) 60-69% | Very good performance which demonstrates the ability to analyse the subject and apply some theory. The work shows a very good level of competence in managing sources and materials and some initiative. Academic writing skills are very good, and expression remains accurate overall. Very good professional skills (where appropriate). The work shows some original thought. |
Lower second (2:2) 50-59% | A good performance which begins to analyse the subject and apply some underpinning theory. The work shows a sound level of competence in managing basic sources and materials. Academic writing skills are good, and expression remains accurate overall although the piece may lack structure. Good professional skills (where appropriate). The work lacks some original thought. |
Third (3) 40-49% | Satisfactory level of performance in which there are some omissions in understanding the subject, its underpinning theory, and ethical considerations. The work shows a satisfactory use of sources and materials. Academic writing skills are limited and there are some errors in expression and the work may lack structure overall. There are some difficulties in developing professional skills (where appropriate). The work lacks original thought and is largely imitative. |
Marginal Fail 30-39% | Limited performance in which there are omissions in understanding the subject, its underpinning theory, and ethical considerations. The work shows a limited use of sources and materials. Academic writing skills are weak and there are errors in expression and the work may lack structure overall. There are difficulties in developing professional skills (where appropriate). The work lacks original thought and is largely imitative. |
Clear fail 29% and Below | A poor performance in which there are substantial gaps in knowledge and understanding, underpinning theory and ethical considerations. The work shows little evidence in the use of appropriate sources and materials. Academic writing skills are very weak and there are numerous errors in expression. The work lacks structure overall. Professional skills (where appropriate) are not developed. The work is imitative. |
Are You Looking for Answer of This Assignment or Essay
The post COM4011 Java Program for Retail Stock Management Coursework appeared first on Students Assignment Help UK.